前言
在 Vue.js 中,v-for
指令用於在模板中渲染列表。它可以輕鬆地根據數組或對象生成多個元素,並支持渲染各種類型的資料結構。
基本語法
v-for
的基本語法如下:
1
| v-for="(item, index) in items"
|
item
:當前迭代的元素。
index
:當前元素的索引(可選)。
items
:要迭代的數組或對象。
搭配 key
使用 v-for
時,必須為每個項目提供唯一的 key
,以便 Vue 更高效地進行 DOM 更新。key
必須是唯一且不重複的值。基本語法如下:
1
| v-for="(item, index) in items" :key="item.id"
|
key
的重要性
- 提升性能:使用
key
可以幫助 Vue 更智能地重用和重新排序元素,從而減少不必要的 DOM 操作。
- 保持狀態:當列表項目包含狀態(如輸入框內容)時,
key
可確保狀態不會丟失。
- 減少重渲染:在列表項目添加、刪除或重新排序時,
key
使更新更精確。
範例
以下是 2 個簡單的範例,資料源分別是 陣列
與 物件
的格式,展示如何使用 v-for
渲染一個水果清單。
陣列用法
1 2 3 4 5 6 7 8 9 10
| <template> <div> <h1>水果清單</h1> <ul> <li v-for="(item, index) in fruits" :key="index"> {{ index + 1 }}:{{ item }} </li> </ul> </div> </template>
|
1 2 3 4 5 6 7 8 9
| <script> export default { data() { return { fruits: ['蘋果', '香蕉', '橙子', '葡萄'] }; } }; </script>
|
1 2 3 4 5 6 7 8 9 10 11 12 13
| <script> import { ref } from 'vue';
export default { setup() { const fruits = ref(['蘋果', '香蕉', '橙子', '葡萄']);
return { fruits, }; } }; </script>
|
1 2 3 4 5 6 7
| <script setup>
import { ref } from 'vue';
const fruits = ref(['蘋果', '香蕉', '橙子', '葡萄']);
</script>
|
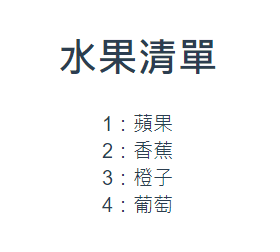
物件用法
1 2 3 4 5 6 7 8 9 10
| <template> <div> <h1>水果清單</h1> <ul> <li v-for="(color, fruit) in fruits" :key="fruit"> {{ fruit }} 是 {{ color }} </li> </ul> </div> </template>
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| <script> export default { data() { return { fruits: { apple: '紅色', banana: '黃色', orange: '橙色', grape: '紫色' } }; } }; </script>
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
| <script> import { reactive } from 'vue';
export default { setup() { const fruits = reactive({ apple: '紅色', banana: '黃色', orange: '橙色', grape: '紫色' });
return { fruits, }; } }; </script>
|
1 2 3 4 5 6 7 8 9 10
| <script setup> import { reactive } from 'vue';
const fruits = reactive({ apple: '紅色', banana: '黃色', orange: '橙色', grape: '紫色' }); </script>
|
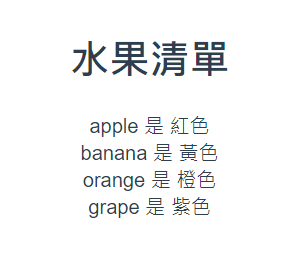
總結
v-for
是 Vue.js 中非常強大的指令,用於動態生成列表。無論是數組還是對象,它都能輕鬆處理,並且能夠有效地跟蹤元素的變化。希望這篇筆記能幫助你更好地理解和使用 v-for
!